Advanced Audience Targeting segment qualification methods for the Swift SDK
Use the Advanced Audience Targeting segment methods to fetch external audience mapping for the user. You can fetch user segments with the user identifier of the user context.
Beta
Advanced Audience Targeting is in beta. Apply on the Optimizely beta signup page or contact your Customer Success Manager.
fetchQualifiedSegments - asynchronous
Minimum SDK version
4.0.0
Description
Fetches all qualified segments for the user context.
fetchQualfiedSegments
is a method of the UserContext
object. See OptimizelyUserContext for details.
Parameters
The following table describes the parameters for the fetchQualifiedSegments
method:
Parameter | Type | Description |
---|---|---|
options (optional) | [OptimizelySegmentOption] | A set of options for fetching qualified segments from ODP. |
completion | (OptimizelyError?) -> Void | A completion handler to be called with the fetch result. |
Returns
The completion handler to be called on completion that updates the qualified segments array.
- On success, it passes a nil error.
- On failure, it passes one of these errors:
OptimizelvError.invalidSegmentidentifier
OptimizelyError.fetchSegmentsFailed
.
Qualified segments array
You can read and write directly to the qualified segments array to bypass the remote fetching process from Optimizely Data Platform (ODP) or utilize your own fetching service. This can be helpful when testing or debugging.
Example fetchQualifiedSegments - asynchronous
The following is an example of calling the fetchQualifiedSegments
method and accessing the returned completion object:
let attributes: [String: Any] = ["app_version": "1.3.2"]
let user = optimizelyClient.createUserContext(userId: "user123", attributes: attributes)
// Without segment option
Task {
let attributes: [String: Any] = ["app_version": "1.3.2"]
let user = optimizely.createUserContext(userId: "user123", attributes: attributes)
try? user.fetchQualifiedSegments()
}
// With segment options
Task {
var odpSegmentOptions: [OptimizelySegmentOption] = [.ignoreCache, .resetCache]
let attributes: [String: Any] = ["app_version": "1.3.2"]
let user = optimizely.createUserContext(userId: "user123", attributes: attributes)
try? user.fetchQualifiedSegments(options: odpSegmentOptions)
}
The SDK fetches the segments then caches them. This means that if the same user is requesting the segments again (when new user contexts are created), you can retrieve the audience segment information from the cache rather than from the remote ODP server.
You can add the following options to your odpSegmentOptions
array to bypass caching:
- ignoreCache – Bypass segments cache for lookup and save.
- resetCache – Reset all segments cache.
fetchQualifiedSegments - synchronous
Minimum SDK version
4.0.0
Description
This is a synchronous version of the fetchQualifiedSegments
asynchronous version above.
Note
This call blocks the calling thread until fetching is completed.
Parameters
The following table describes the parameters for the fetchQualifiedSegments
method:
Parameter | Type | Description |
---|---|---|
options (optional) | [OptimizelySegmentOption] | A set of options for fetching qualified segments from ODP. |
Returns
This method does not provide a return value. If it fails, it throws one of these errors:
OptimizelyError.invalidSegmentIdentifier
OptimizelyError-fetchSegmentsFailed(String)
Example fetchQualifiedSegments - synchronous
The following is an example of calling the fetchQualifiedSegments
method and accessing the returned completion object:
let optimizely = OptimizelyClient(sdkKey: <Your_SDK_Key>)
optimizely.start { result in
let attributes: [String: Any] = ("app version": "1.3.2")
let user = optimizelyClient.createUserContext(userld: "user123", attributes: attributes)
do {
// this will block the calling thread until fetch is completed.
try user.fetchQualifiedSegments()
let decision = user. decide(key: "flag1")
} catch OptimizelyError.invalidSeqmentIdentifier {
print("[AudienceSegments] audience segments fetch failed (user id is not reoistered yet or invalid)")
} catch {
print("[AudienceSegments] \(error)")
}
}
The following diagram shows the network calls between your application, the Swift SDK, and the ODP server when calling fetchQualifiedSegments
:
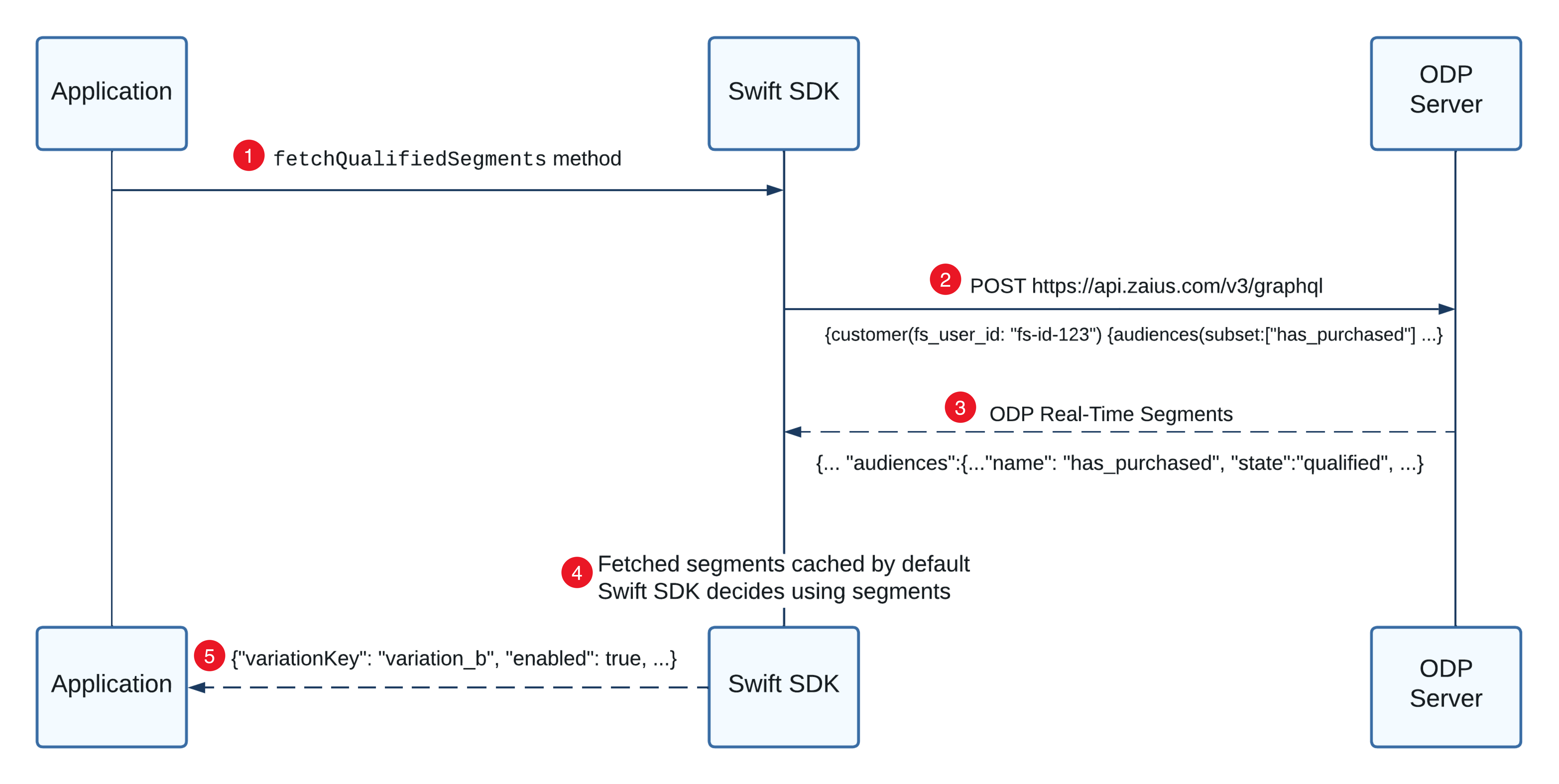
- Call
fetchQualifiedSegments
method. - Swift SDK makes GraphQL call to ODP to fetch segments.
- ODP responds with segments.
- Fetched segments mapping user IDs to segments are cached.
- Appropriate variations are returned for user.
isQualifiedFor
Minimum SDK version
4.0.0
Description
Check if the user qualifies for the given audience segment.
Parameters
The following table describes the parameters for the isQualifiedFor
method:
Parameter | Type | Description |
---|---|---|
segment | String | The ODP audience segment name to check if the user qualifies |
Returns
true
if the user is qualified.
Examples
The following is an example of whether or not the user qualifies for an ODP segment:
let attributes: [String: Any] = ["laptop_os": "mac"]
let user = optimizelyClient.createUserContext(userId: "user123", attributes: attributes)
user.fetchQualifiedSegments { segments, err in
let isQualified = user.isQualifiedFor(segment: "segment1")
}
Source files
The language and platform source files containing the implementation for Swift are available on Github.
Updated 3 months ago