Consume data with queries
How to run queries on data from external sources.
After Defining the content types and Synchronizing your data to Optimizely Graph, you can start consuming it with GraphQL queries and build a simple web application.
Note
This tutorial uses the non-commercial datasets of IMDb.
Creating GraphQL queries
We can start with a simple query first. This one lists the first authors that were synchronized:
{
Actor {
total
items {
knownForTitles
primaryName
}
}
}
However, because the IMDb data is very normalized, it makes sense to create single Optimizely Graph queries with the joins with linking. The following example shows that you can join the data from all 3 datasets with a single query and get a single view. Here we get information from an actor, and then for each actor, the titles that the person was in, and then for each title the rating.
query MyQuery {
Actor (
orderBy: { _ranking: SEMANTIC }
where: { _fulltext: { match: "kung fu" }
){
total(all: true)
items {
primaryName
primaryProfession
_link (type:DEFAULT) {
Title (orderBy: {primaryTitle: ASC}) {
items {
primaryTitle
genres
_link(type:TITLES) {
Rating {
items {
averageRating
numVotes
}
}
}
}
}
}
}
}
}
And we can present another view on the data as well by ordering by rating and as example, only get the titles that are rated 9 or lower, and then for each rating, the titles, and then for each title, and all the persons involved in the title.
query MyQuery {
Rating(
where: { averageRating: { lte: 9 } }
orderBy: { averageRating: DESC, numVotes: DESC }
) {
total (all: true)
items {
tconst
numVotes
averageRating
linkWithDefaultType: _link(type: TITLES) {
Title {
items {
primaryTitle
originalTitle
genres
tconst
_fulltext
}
}
}
linkWithTitleToActor: _link(type: TITLE_TO_ACTOR) {
Actor {
items {
primaryName
_link (type: DEFAULT) {
Title {
items {
primaryTitle
genres
}
}
}
}
}
}
}
}
}
We can combine the different views on Actor
and Title
into a single query with inline fragments.
{
Record(
limit: 5
where: { _fulltext: { match: "fred astaire" } }
orderBy: { _ranking: SEMANTIC }
) {
total
items {
__typename
... on Title {
primaryTitle
genres
actors: _link(type: TITLE_TO_ACTOR) {
Actor {
items {
primaryName
primaryProfession
birthYear
deathYear
}
}
}
ratings: _link(type: TITLES) {
Rating {
items {
averageRating
numVotes
}
}
}
}
... on Actor {
primaryName
_link(type: DEFAULT) {
Title {
items {
primaryTitle
genres
_link(type: TITLES) {
Rating {
items {
averageRating
numVotes
tconst
}
}
}
}
}
}
}
}
}
}
Creating views in a web application
You can use the following GraphQL queries as query templates to build a site. To show how easy it is to build a site with site search support, Optimizely has created this simple demo application that allows you to build a site with Python, Flask, and Optimizely Graph. All other code is available on GitHub, such as sample data and ingestion script has been included as well.
Note
Optimizely has selected a subset of the datasets to demo. Additionally, Optimizely has ignored the design of the webpage and instead focused on demonstrating the state-of-the-art search capability.
This example shows the results of listing the first 20 actors with the GraphQL query listed previously, including information and their titles and ratings.
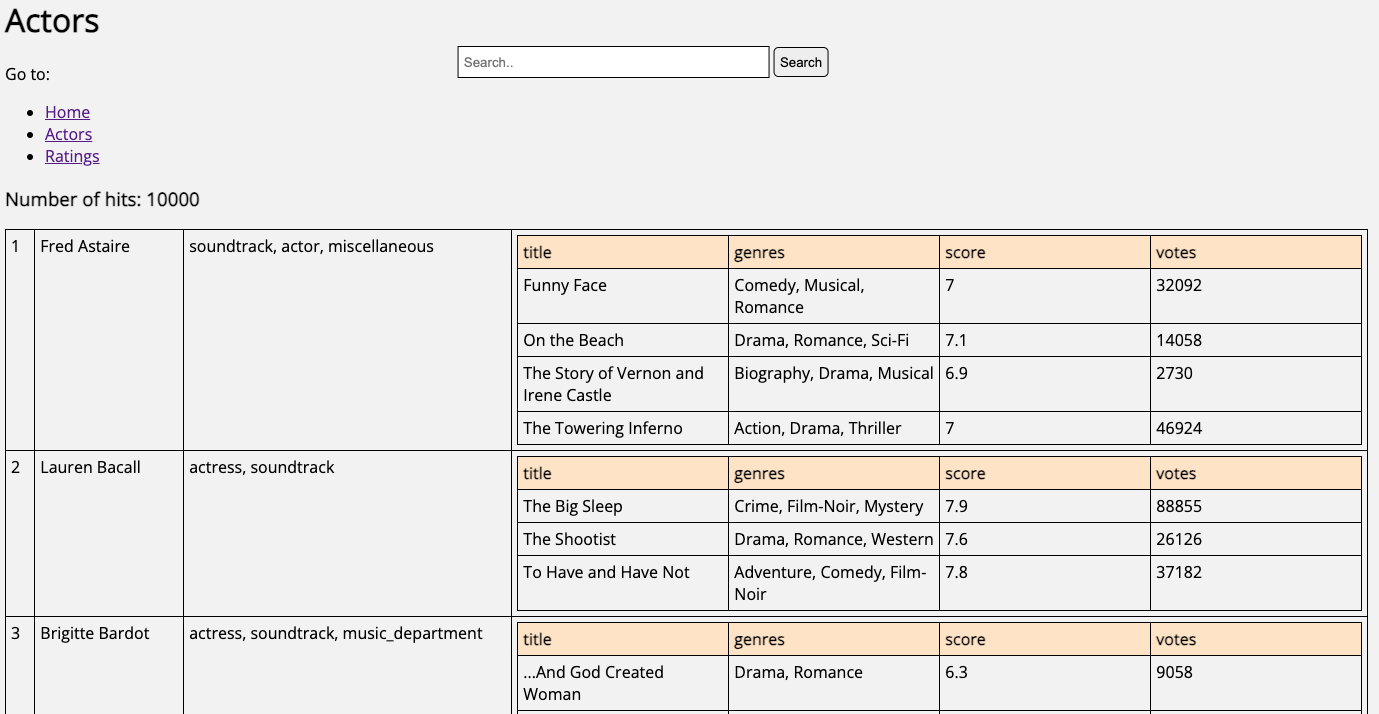
You can run queries with Semantic search. For example, searching for "kung fu" and returned 2 names which can be considered as relevant.
Note
For this tutorial, all of the IMDb datasets have not been synchronized. The results in this tutorial is not complete and could change as more data is synchronized.
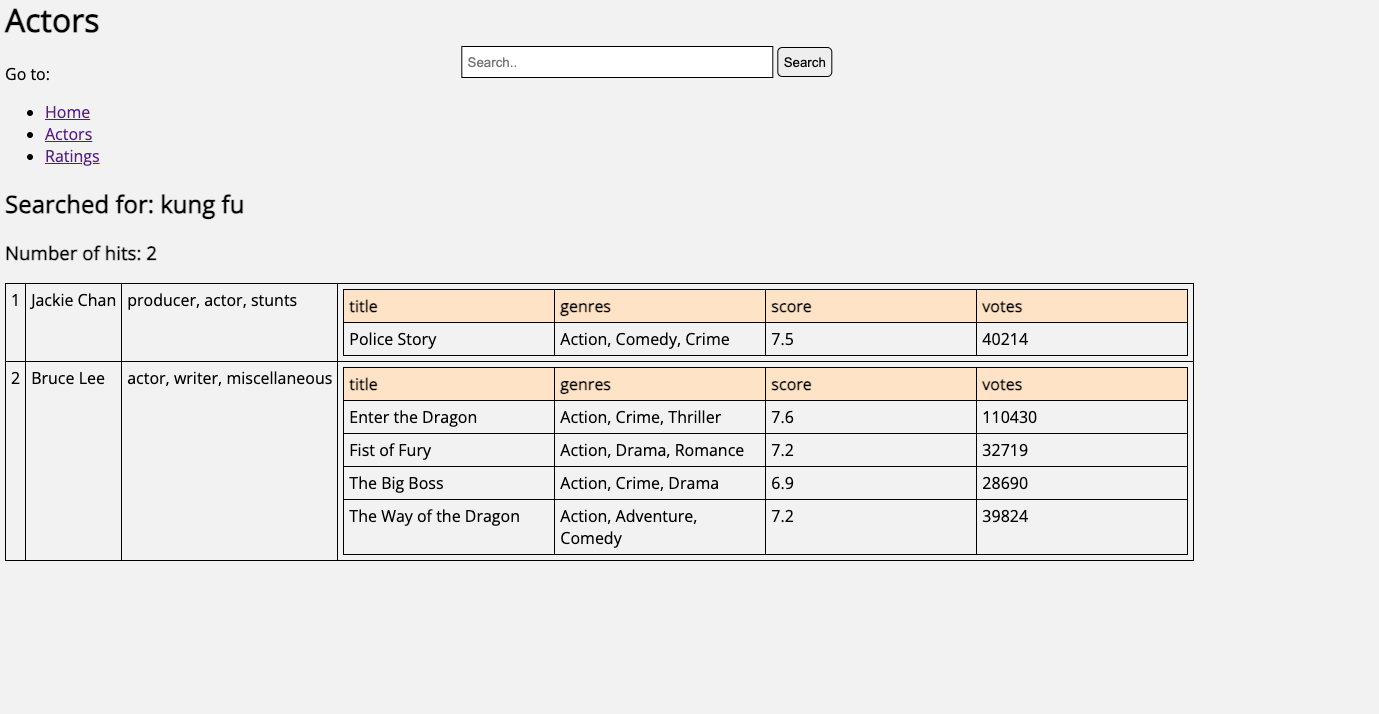
You can present a totally different view of the same data. For example, by rating.
Note
For this tutorial, all of the IMDb datasets have not been synchronized, so the title is missing in the example. But, given the other information, could you guess the title?
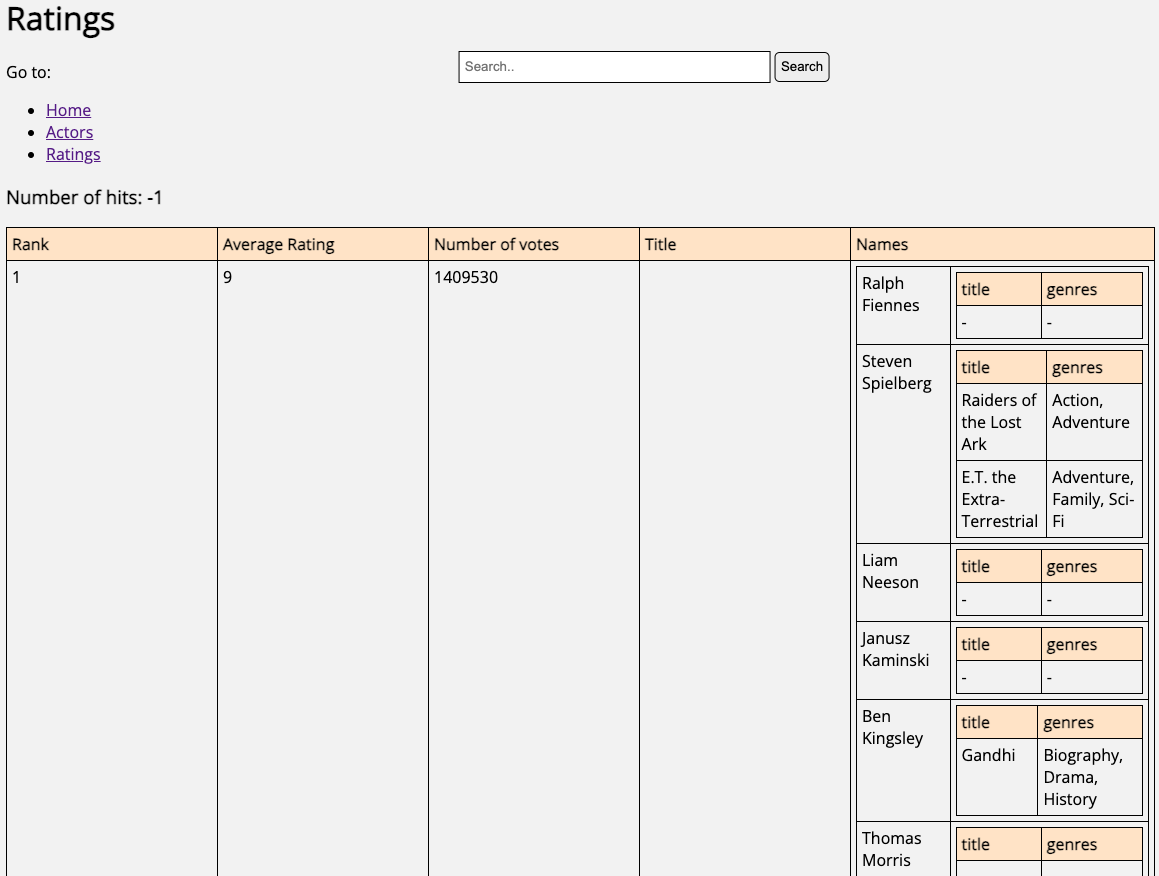
In the following image, you see that "The Godfather Part II" has an average rating of 9, over 1.3 million votes, the people involved in the movie production, and then per person the titles and genres they were involved in. The powerful ability to join your data as a graph explains why the service is called Optimizely Graph.
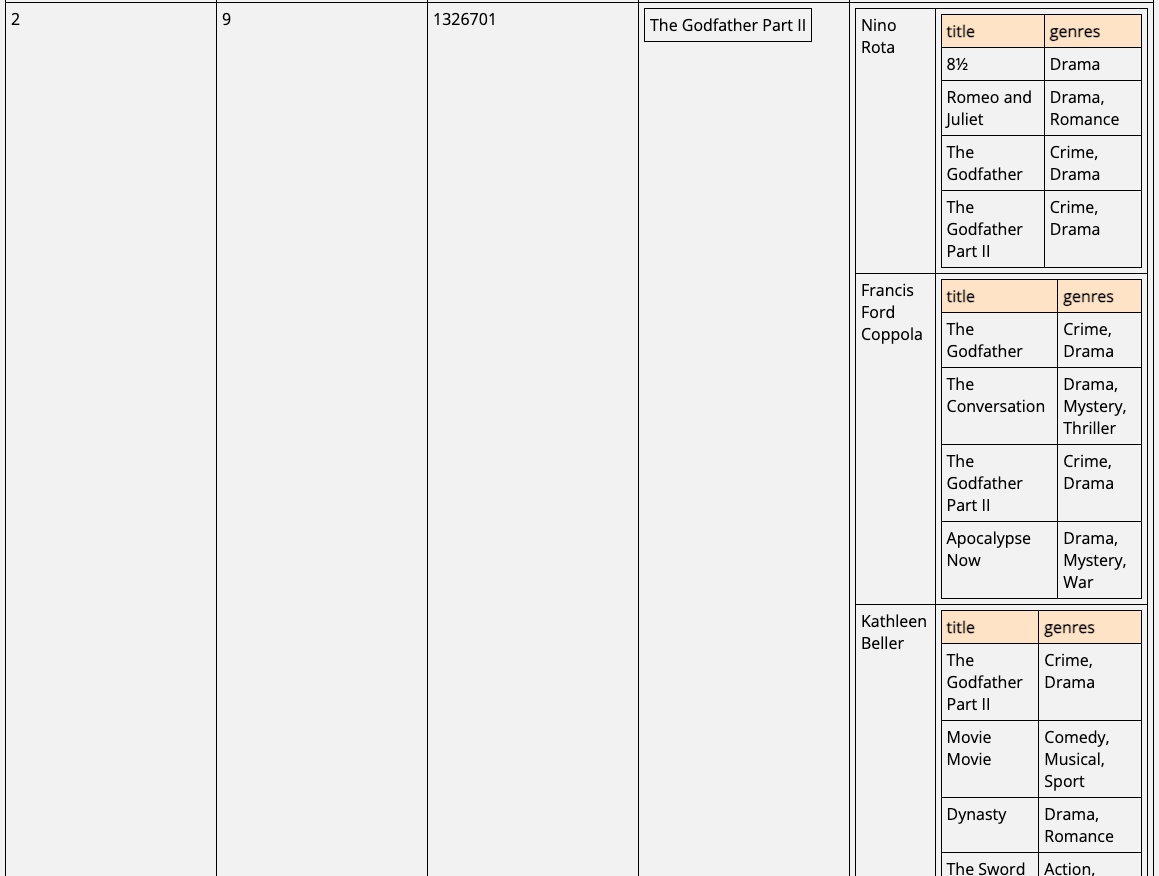
Updated 23 days ago